Creating interactive infographics with plain Javascript (Part-three)
Recap
This article is a five-part series on creating interactive infographics with plain Javascript.
Previously we added navigation features to interact with content. We can scroll, zoom, pan, or drag a large canvas any way we like.
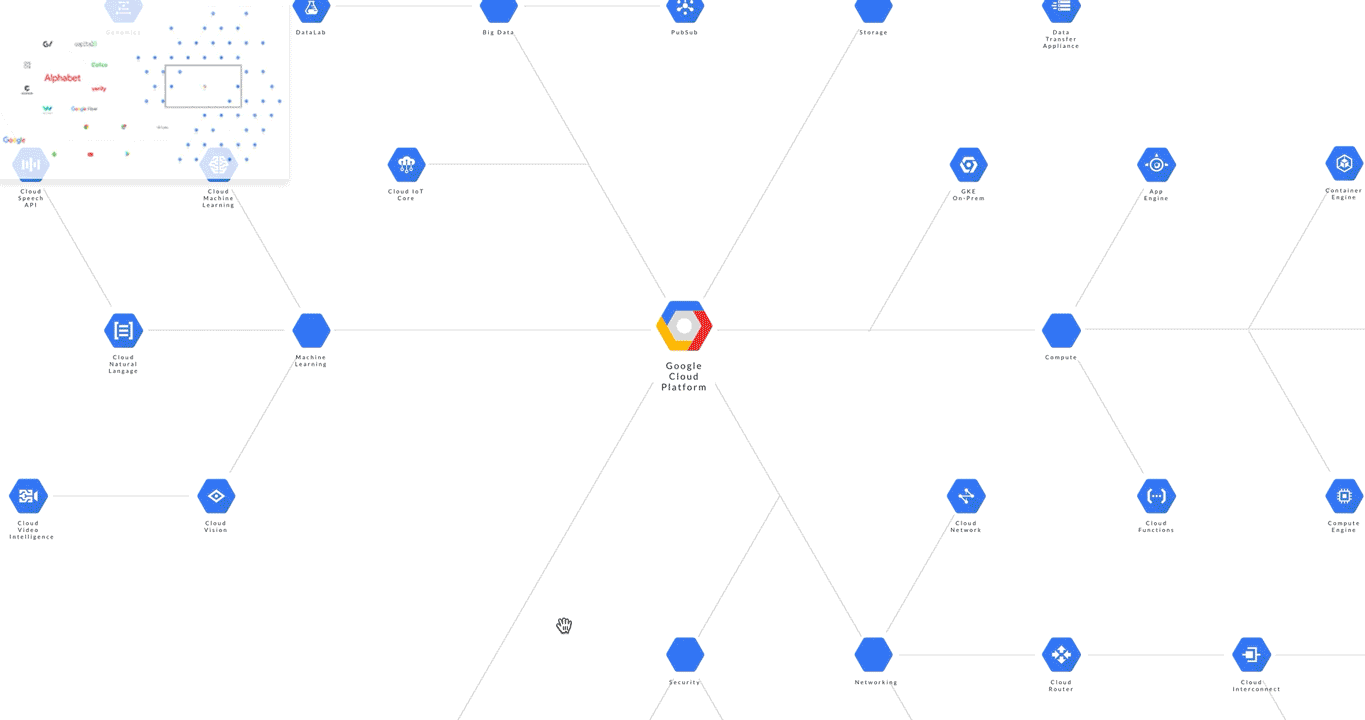
Did you notice something special? There’s a mini-map that responds to user actions. What’s going on?
Objective
We are going to add a map locator to enhance navigation.
Introduction
Map locators are useful for exploring large canvases. It is a simple yet effective visualisation gadget that provides continuous updates on the current locations. The gadget is also ideal for illustrating thematic relationships among interacting components.
Concept
The map locator comprises a miniMap
and a location indicator
. A miniMap is a scaled-down version of the actual-sized canvas
. The location indicator is an icon or a marker that states the user’s current location.
You can use the map locator in two ways:
- When visitors scroll/zoom/pan/drag the canvas, the indicator automatically moves to its new position. You’ve seen that in action at the earlier intro/recap demo video.
- Conversely, visitors can also use the indicator itself to interact with the canvas. You can either drag the indicator or point-and-click on any areas to navigate to any new regions.
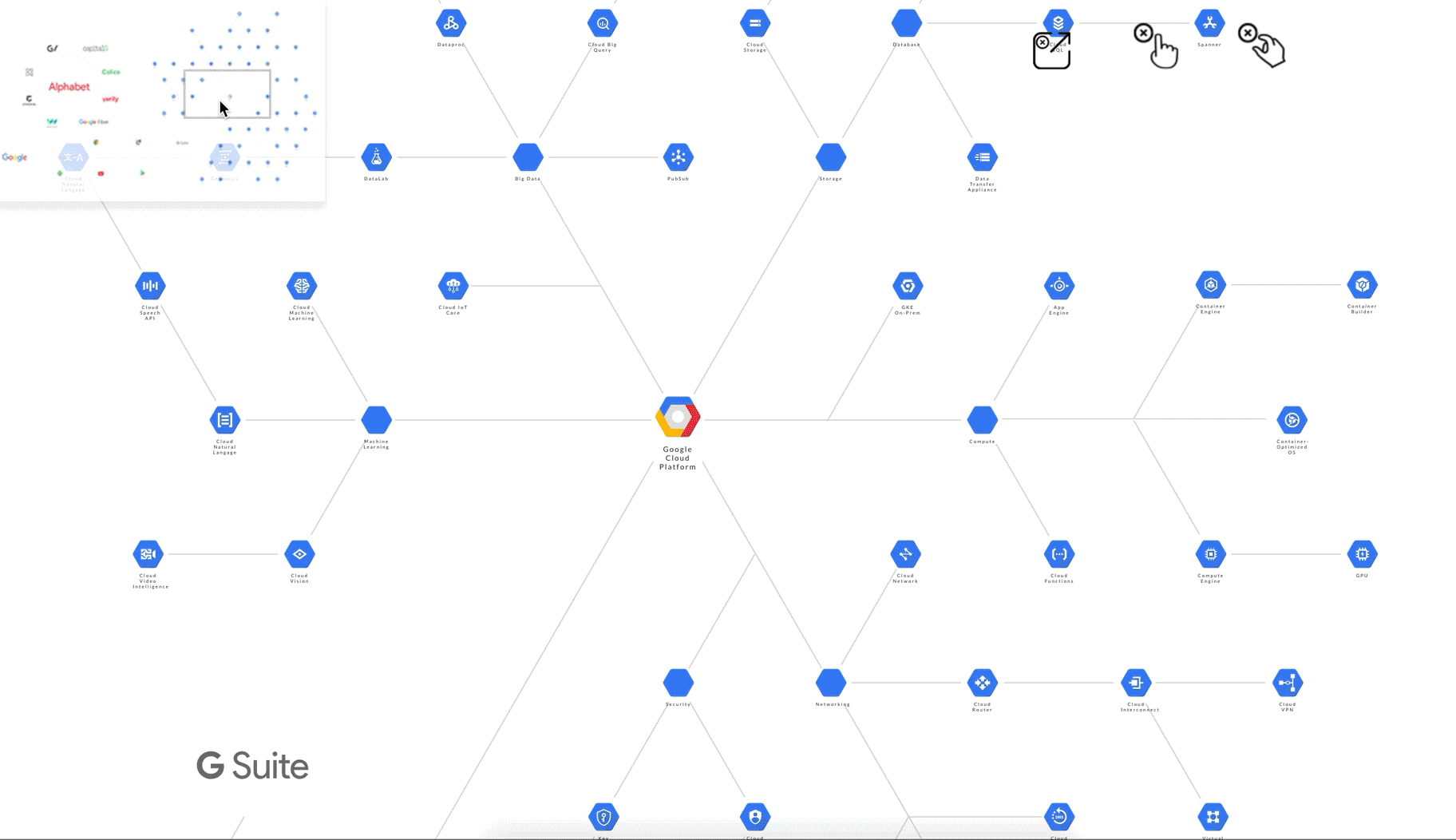
Getting started — the concept of action and reaction
Recall from the part-two discussion that we have a concept of action and reaction for our UI? In gist, an action on a mouse produces an equal reactionon an HTML element. For example, a cursor movement causes the canvas to move by the same distance. This mirroring effect is measured as the mouse delta value.
We’ll apply the same concept to build the map locator. A delta movement of the location indicator translates into a new relative scroll value on a bigger canvas. We can map this both ways to create a versatile two-way navigation gadget.
Prepping the miniMap container
Create a reference to the miniMap
container. It will stay on top of the canvas to guide navigation.
var miniMap = document.getElementById( “miniMap” );
Create a reference indicator
that exists inside the miniMap
container.
var indicator = document.getElementById( "indicator" );
Use any method to generate your miniMap. We’ll take a shortcut and use a scaled down static image of the canvas.
<img src=”myMap.jpg” id=”miniMap”>
Remember to use z-index
to set the correct order of appearance.
Navigating with the indicator
Add an event listener to capture the mouse delta value of the indicator
and update the new relative scroll position of the canvas
.
miniMap.addEventListener("mousedown", handlerIndicator, false);
function handlerIndicator(event) { if (event.which == 1) { // formula } }
The formula should dynamically update the canvas scroll value based on the relative mouse delta value:
canvas.scrollTo( ( canvas.scrollWidth * (event.clientX - boundingbox(0, 0, miniMap).x) / boundingbox(0, 0, miniMap).w ) - canvas.offsetWidth/2, (canvas.scrollHeight * (event.clientY - boundingbox(0, 0, miniMap).y) / boundingbox(0, 0, miniMap).h ) - canvas.offsetHeight/2 );
canvas.scrollTo
tells the browser to execute a scroll whenever amousedown
event on theminiMap
is detected.event.clientX
andevent.clientY
returns the X and Y coordinates of the mouse cursor. These values are dynamically updated by the browser engine.scrollWidth
returns the width of the canvas (i.e. the parent container).event.clientX — boundingbox(0, 0, miniMap).x) / boundingbox(0, 0, miniMap).w
translates the relative indicator movement onto the actual-sized canvas.canvas.offsetWidth/2
andcanvas.offsetHeight/2
initiates the correct scroll offset values.
We have created a one-way navigation aid. Let’s make it two-way.
Navigating the canvas
Create an event listener to call a custom function handler_mouseScroll
whenever interaction is detected on the canvas (i.e. scroll/pan/drag)
canvas.addEventListener("scroll", handlerMouseScroll, false);
function handlerMouseScroll(event) { var scrollpercentX = canvas.scrollLeft / canvas.scrollWidth;
var scrollpercentY = canvas.scrollTop / canvas.scrollHeight;
indicator.style.left = miniMap.offsetWidth * scrollpercentx + "px"; indicator.style.top = miniMap.offsetHeight * scrollpercenty + "px"; ... }
scrollpercentX
andscrollpercentY
gives the conversion ratio for calculating the relative scroll values on the mini-map.miniMap.offsetWidth
andminiMap.offsetHeight
gives the dimensions of the miniMap.indicator.style.left
andindicator.style.top
updates the position of the indicator on the mini map.
Next Steps
With a two-way navigation gadget, visitors can explore a large canvas without getting disoriented. We can create infographics that are sophisticated yet easy to use.
In the next article, we’ll design a UI that can represent the information architecture of multifaceted content.
SOURCE: https://hackernoon.com/how-to-create-a-two-way-navigation-map-e96600a73cbd
WRITE BY