There are many arguments on the web whether using a CSS Preprocessor is useful in development or not. My personal opinion is that you may not need a preprocessor for small web projects or applications.
However, maintenance & readability of CSS code gets harder as the project gets bigger. Dealing with thousands of lines of CSS rules, waste developers time and raise the cost of the project. As the project gets bigger, CSS causes some problems like:
- Big effort for little changes
- Difficulties in structuring the code
- Code redundancy
- Endless lines of CSS classes & rules
A preprocessor helps us to deal with these problems. It has some advantages over regular CSS. Before we dive deeper, first explain what a CSS preprocessor is…
What is a CSS Preprocessor?
A program/tool that has its own syntax which gets compiled later in standard CSS code.
A preprocessor has its own syntax for developers to write easier and cleaner CSS code. Later, it gets translated in a separate file to standard CSS, because browsers don’t understand the syntax.
There are different preprocessors like Sass, Less, and Stylus. In this article, I will be explaining some advantages of Sass.
What is Sass?
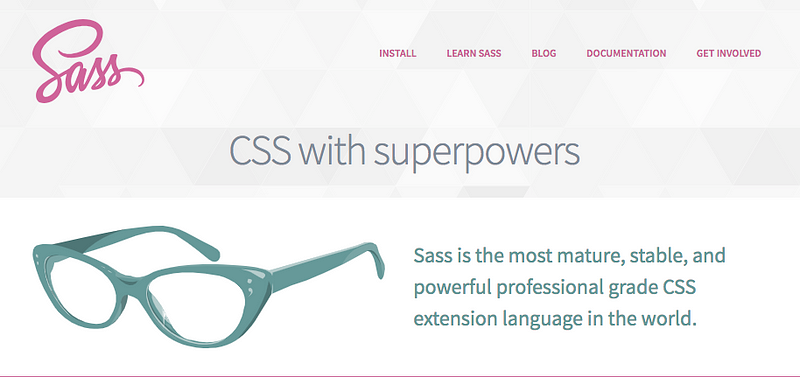
Sass is one of the most widely used CSS Preprocessors. It has various features to help developers write better and cleaner CSS code. You can check for more details from Sass official website & Github repository.
FAQ: Sass vs SCSS
This is a commonly asked question. They are actually both Sass with a different syntax. SCSS is basically a newer version, Sass Version 3.
Example of Sass syntax:
$color: gray
=my-font($color
) font-family: Arial, Helvetica, sans-serif font-size: 16px color: $color
body
background: $color
margin: 0
+my-font(white)
Example of SCSS syntax:
$color: gray;
@mixin my-font($color) { font-family: Arial, Helvetica, sans-serif; font-size: 16px; color: $color; }
body {
background: $color;
margin: 0;
@include my-font(white);
}
As we can see, SCSS (Sassy CSS) has a CSS-like syntax, which is much easier to read. It is an extension of CSS, whereas Sass has a more different syntax. Their file extension is also different: .sass
& .scss
.
You can read more about it here. Let’s move on with the features of Sass.
Feature #1: Variables
Various CSS classes can contain the same rule or rules in a project. For example, we have 20 boxes on our webpage with different background colors:
.box-1 { width: 100px; height: 100px; background: red; }
.box-2 { width: 100px; height: 100px; background: yellow; }
...
.box-20 { width: 100px; height: 100px; background: blue; }
Later, our client changes mind and wants bigger boxes. So I need to increase every class’s width
and height
properties one by one. This could also be 50 classes. In real life programming, this can be frustrating. As I mentioned above, this is an example of big effort for little changes.
How can we do it more efficiently?
Sass provides a solution: variables. Like in other programming languages, we can use variables to store values and reuse them later.
Definition of a variable:
$variable-name: value;
Going back to example above, if we define variables for width
& height
:
$box-width: 100px; $box-height: 100px;
later when a change needed, only we have to do is to change their values once:
$box-width: 200px; // changed from 100px to 200px $box-height: 200px; // that's all!
.box-1 { width: $box-width; // using variables now instead of pixels height: $box-height; background: red; }
.box-2 { width: $box-width; height: $box-height; background: yellow; }
...
.box-20 { width: $box-width; height: $box-height; background: blue; }
CSS itself also supports variables now, but it doesn’t work in IE & old versions of other browsers:
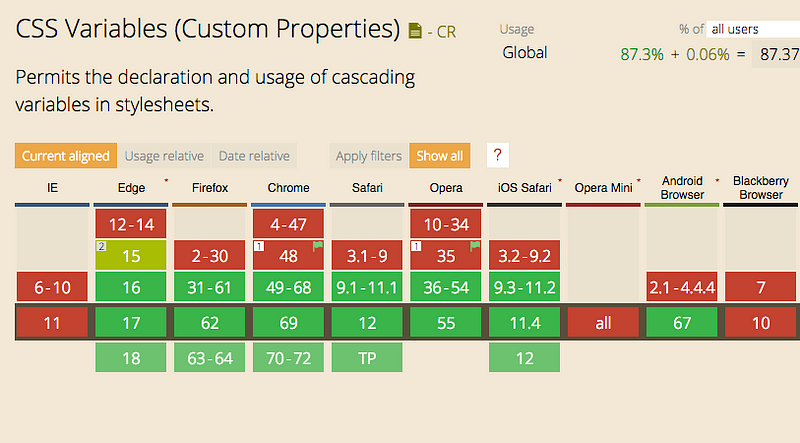
Feature #2: Nesting
Standard CSS doesn’t support nesting. We can’t write a class inside another class. As the project gets bigger, this brings a readability problem and the structure doesn’t look nice.
For example, let’s define a navigation menu with clickable links in HTML below:
<nav> <ul> <li><a href="#">Home</a></li> <li><a href="/about">About</a></li> <li><a href="/contact">Contact</a></li> </ul> </nav>
HTML supports nested code. However without nesting, they look like this in CSS:
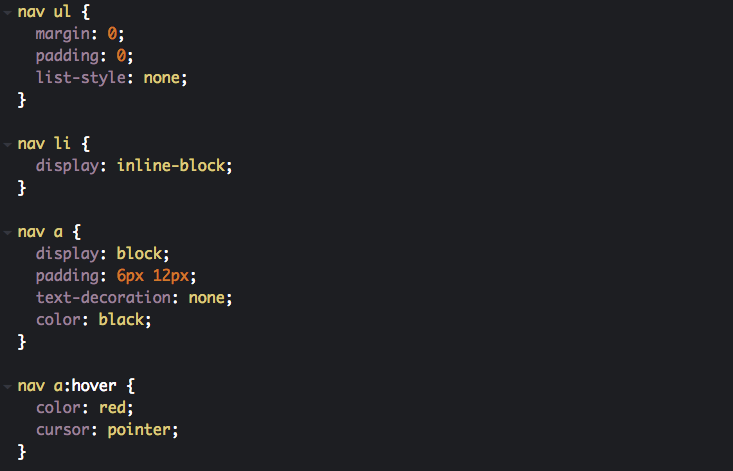
We had to write nav for each tag, even for the pseudo-class of the anchor (hover) because nav is the parent tag of all. Sass however, supports nesting:
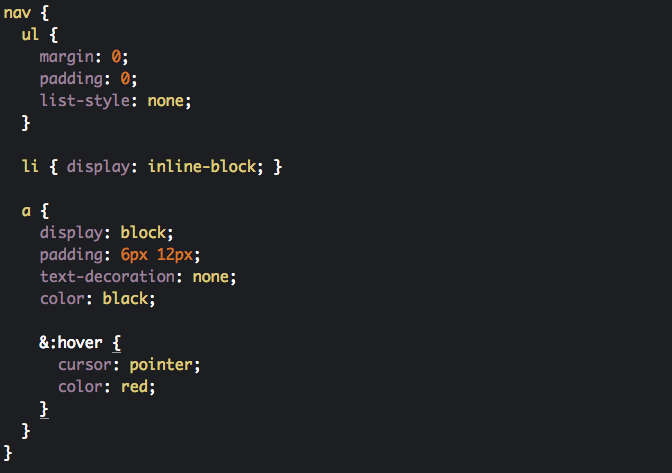
Here we can write better structured code like in HTML. We don’t need to write nav
class after class, which also prevents redundancy
Important: It is not recommended to nest classes deeper than 3 levels.
Feature #3: Mixins
We learned above how to use variables for CSS rules. But what if we need to use a group of rules together? Sass has a feature called mixins, letting us do it.
What is a Mixin?
Mixins are Sass functions that group CSS declarations together. We can reuse them later like variables.
We can create a mixin with @ mixin command, followed by a name:
@mixin my-font { font-family: Arial, Helvetica, sans-serif; font-size: 16px; font-style: italic; }
or we can create a mixin as a function and add parameters as well:
$font-color: red;
@mixin my-font($font-color) { font-family: Arial, Helvetica, sans-serif; font-size: 16px; font-style: italic; color: $font-color; }
After creating the mixin, we can use it in any class with @ include command. So we can use the my-font mixin instead of 4 lines of font rules each time. This approach simplifies the code.
p {
@include my-font;
}
Using mixins is a good way to prevent code redundancy.
Feature #4: Imports
Finally, we can cut our huge CSS files into smaller pieces with Sass importfeature. It is much easier to read & maintain smaller files rather than one big file with endless lines.
Actually, CSS also has now an import feature. But it works differently. CSS sends an HTTP request to server each time to import a file. Sass does it without an HTTP request, which is a faster approach.
All you need to is, import your Sass file with @ import command to another Sass file:
// Your main Sass file
@import 'file';
@import 'anotherFile';
.class {
// Your code
}
We don’t have to use the .scss extensions in file path, Sass will understand it.
So these are some important features of Sass, which helps us to write more efficient CSS code. There are also other nice features, maybe I can cover them in another article. For a better understanding, you can install Sass to your system. Or you can directly start coding at codepen.io.
I hope you find this article helpful. If there is something you don’t understand, comment your questions below.
Check my profile for other articles:
Thank you very much & till next time!
SOURCE: https://medium.com/swlh/advantages-of-using-a-preprocessor-sass-in-css-eb7310179944
Written by
Cem Eygi
Front-end Developer
The Startup
Medium’s largest publication for makers. Subscribe to receive our top stories here → https://goo.gl/zHcLJi