Pure functions are the atomic building blocks in functional programming. They are adored for their simplicity and testability.
This post covers a quick checklist to tell if a function’s pure or not.
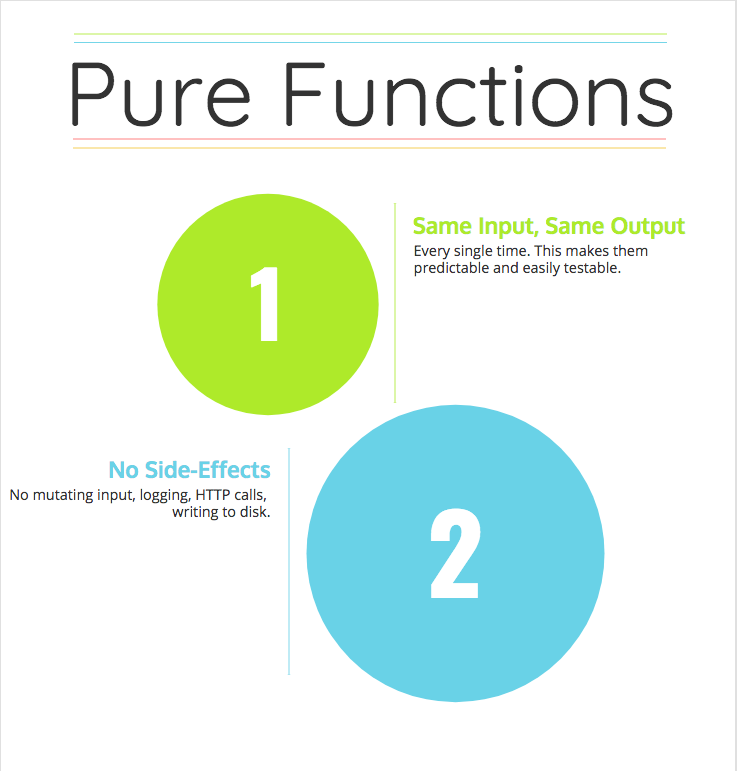
The Checklist
A function must pass two tests to be considered “pure”:
-
- Same inputs always return same outputs
- No side-effects
Let’s zoom in on each one.
1. Same Input => Same Output
Compare this:
const add = (x, y) => x + y;
add(2, 4); // 6
To this:
let x = 2;
const add = (y) => { x += y; };
add(4); // x === 6 (the first time)
Pure Functions = Consistent Results
The first example returns a value based on the given parameters, regardless of where/when you call it.
If you pass 2
and 4
, you’ll always get 6
.
Nothing else affects the output.
Impure Functions = Inconsistent Results
The second example returns nothing. It relies on shared state to do its job by incrementing a variable outside of its own scope.
This pattern is a developer’s nightmare fuel.
Shared state introduces a time dependency. You get different results depending on when you called the function. The first time results in 6
, next time is 10
and so on.
Which Version’s Easier to Reason About?
Which one’s less likely to breed bugs that happen only under certain conditions?
Which one’s more likely to succeed in a multi-threaded environment where time dependencies can break the system?
Definitely the first one.
2. No Side-Effects
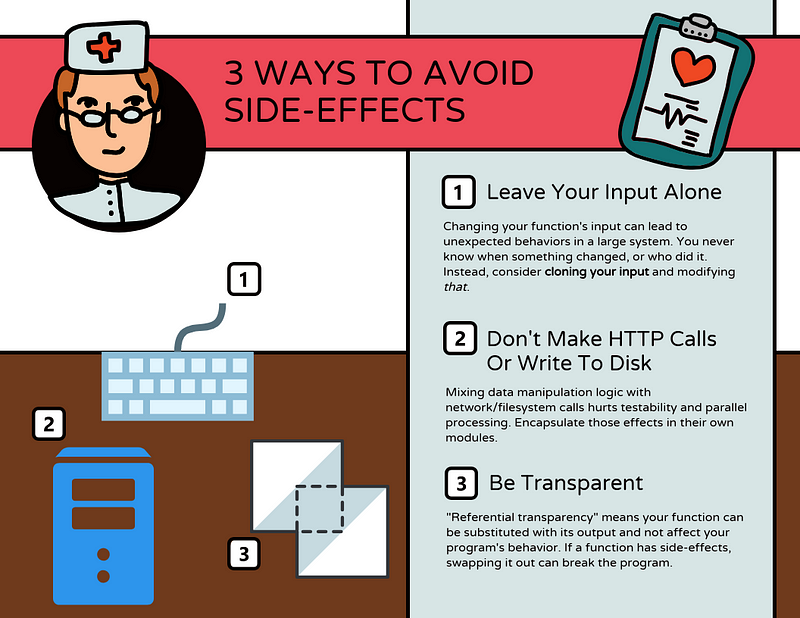
This test itself is a checklist. A few examples of side-effects are
-
- Mutating your input
-
console.log
-
- HTTP calls (AJAX/fetch)
-
- Changing the filesystem (fs)
- Querying the DOM
Basically any work a function performs that isn’t related to calculating the final output.
I recommend watching this snippet by Uncle Bob Martin on the problem of state. It starts around 15 min.
Here’s an impure function with a side-effect.
Not So Bad
const impureDouble = (x) => { console.log('doubling', x);
return x * 2; };
const result = impureDouble(4);
console.log({ result });
console.log
is the side-effect here but, in all practicality, it won’t harm us. We’ll still get the same outputs, given the same inputs.
This, however, may cause a problem.
“Impurely” Changing an Object
const impureAssoc = (key, value, object) => { object[key] = value; };
const person = { name: 'Bobo' };
const result = impureAssoc('shoeSize', 400, person);
console.log({ person, result });
The variable, person
, has been forever changed because our function introduced an assignment statement.
Shared state means impureAssoc
‘s impact isn’t fully obvious anymore. Understanding its effect on a system now involves tracking down every variable it’s ever touched and knowing their histories.
Shared state = timing dependencies.
We can purify impureAssoc
by simply returning a new object with our desired properties.
Purifying It Up
const pureAssoc = (key, value, object) => ({ ...object, [key]: value });
const person = { name: 'Bobo' };
const result = pureAssoc('shoeSize', 400, person);
console.log({ person, result });
Now pureAssoc
returns a testable result and we’ll never worry if it quietly mutated something elsewhere.
You could even do the following and remain pure:
Another Pure Way
const pureAssoc = (key, value, object) => { const newObject = { ...object };
newObject[key] = value;
return newObject; };
const person = { name: 'Bobo' };
const result = pureAssoc('shoeSize', 400, person);
console.log({ person, result });
Mutating your input can be dangerous, but mutating a copy of it is no problem. Our end result is still a testable, predictable function that works no matter where/when you call it.
The mutation’s limited to that small scope and you’re still returning a value.
Summary
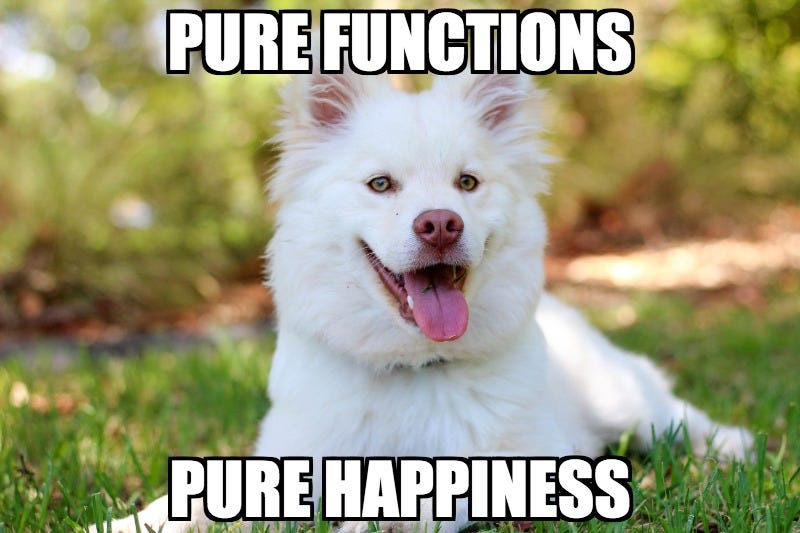
-
- A function’s pure if it’s free from side-effects and returns the same output, given the same input.
-
- Side-effects include: mutating input, HTTP calls, writing to disk, printing to the screen.
-
- You can safely clone, then mutate, your input. Just leave the original one untouched.
- Spread syntax (
…
syntax) is the easiest way to clone objects and arrays.
My Free Course
This tutorial was from my completely free course on Educative.io, Functional Programming Patterns With RamdaJS!
Please consider taking/sharing it if you enjoyed this content.
It’s full of lessons, graphics, exercises, and runnable code samples to teach you a basic functional programming style using RamdaJS.
I’m also on Twitter if you’d like to talk. Until next time!
Take care,
Yazeed Bzadough
http://yazeedb.com/
Source: https://medium.freecodecamp.org/what-is-a-pure-function-in-javascript-acb887375dfe
Written by
freeCodeCamp.org
Stories worth reading about programming and technology from our open source community.