Truth can only be found in one place: the code.
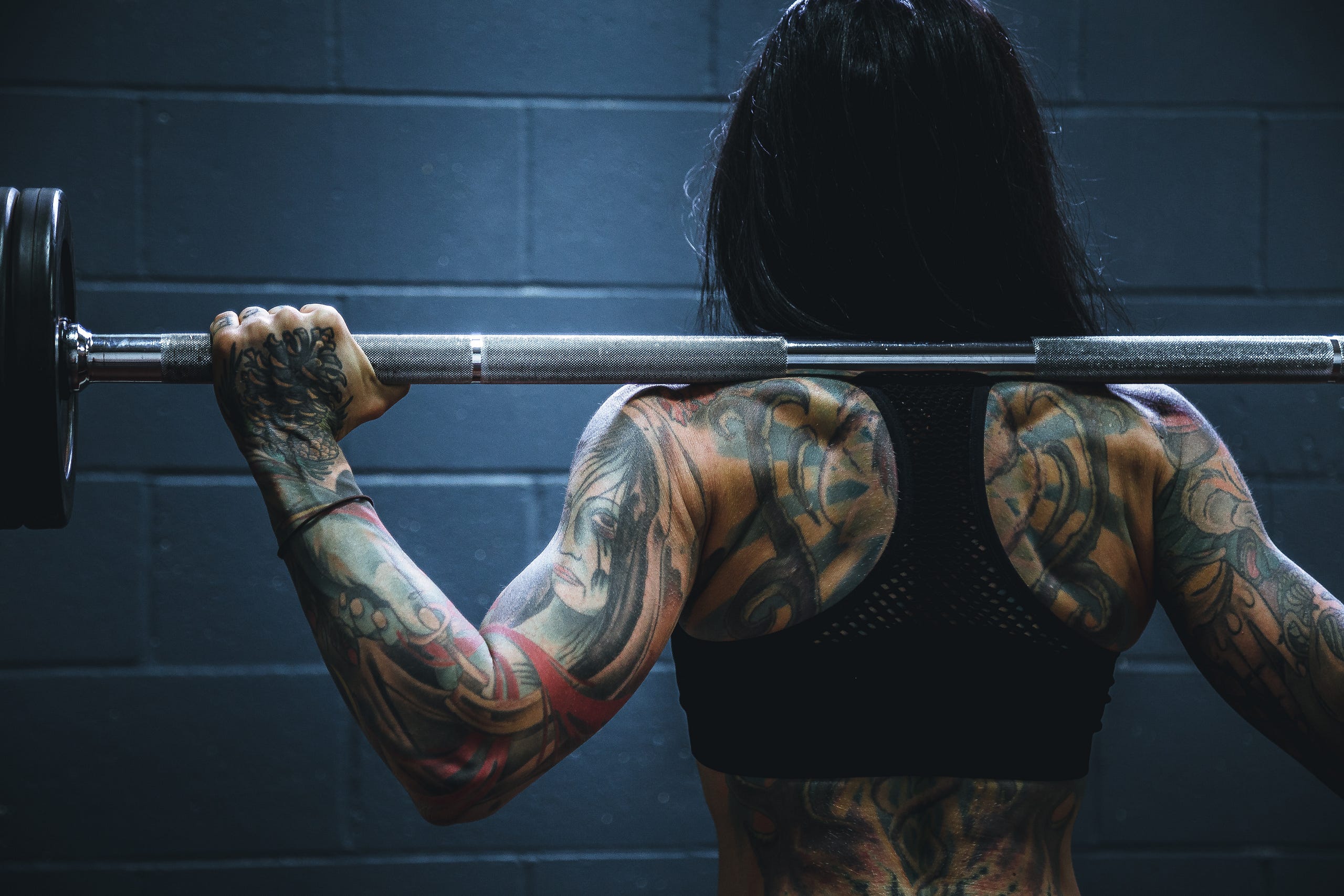
In eXtreme Programming terms, this technique is called “programming by intention” and this is one of the most effective ways to write robust code.
Programming by intention works on the natural ability of the brain to learn any programming language. Whenever we learn any language, the natural tendency is to break down the problems into a series of functional steps. The more micro steps you break, the more you get into the depth of the language. This technique precisely capitalizes on that.
Programming by Intention says, rather than actually writing the code in each case, instead pretend that you already have an ideal method, local in scope to your current object, that does precisely what you want. Ask yourself, “What parameters would such an ideal method take, and what would it return? And, what name would make the most sense to me, right now, as I imagine this method already exists?”
And since the method does not actually exist, you are not constrained by anything other than your intentions.
Consider the code snippet below.
REPORT ysdnblog_class_ideal.
parameters : p_rows type count default ‘100’.
* — — — — — — — — — — — — — — — — — — — — — — — -*
* CLASS lcl_main DEFINITION
* — — — — — — — — — — — — — — — — — — — — — — — -*
CLASS lcl_main DEFINITION.
PUBLIC SECTION.
CLASS-METHODS : start.
PRIVATE SECTION.
METHODS : get_data ,
display.
CLASS-DATA : lr_main TYPE REF TO lcl_main.
DATA it_mara TYPE mara_tt.
ENDCLASS. “lcl_main DEFINITION
* — — — — — — — — — — — — — — — — — — — — — — — -*
* CLASS lcl_main IMPLEMENTATION
* — — — — — — — — — — — — — — — — — — — — — — — -*
CLASS lcl_main IMPLEMENTATION.
METHOD start.
CREATE OBJECT lr_main.
lr_main->get_data( ).
lr_main->display( ).
ENDMETHOD. “start
METHOD get_data.
— — WRITE SOME CODE HERE……
ENDMETHOD. “GET_DATA
METHOD display.
— — WRITE SOME CODE HERE……
ENDMETHOD. “display
ENDCLASS. “lcl_main IMPLEMENTATION
START-OF-SELECTION.
lcl_main=>start( ).
The start() is the public method of the class which is invoked while executing the report. The other methods get_data() and display() are private methods of the class which are invoked locally by instantiating an object of the class.
We can simply call these methods as functional steps. The point is that their existence is part of the internal implementation of this class, not part of the way it is used from the outside.
And they do not exist yet. So when you try to compile this code, the compiler will throw an error stating they do not exist. They have to be created for the code to be compiled.
That is precisely what we must do next. So by programming by intention, we allow ourselves to focus purely on how we are breaking down the problem into the overall context of the requirement. We are not writing one big black chunk of code upfront and executing. All we are doing here is breaking down the code into functional steps and then creating the steps one by one.
Consider this code snippet below in which I wrote the code for the functional steps get_data() and display().
METHOD get_data.
SELECT * FROM mara INTO TABLE me->it_mara UP TO P_rows ROWS .
ENDMETHOD. “GET_DATA
METHOD display.
DATA : lr_table TYPE REF TO cl_salv_table.
cl_salv_table=>factory( IMPORTING r_salv_table = lr_table
CHANGING t_table = me->it_mara ) .
lr_table->display( ).
ENDMETHOD. “display
There is no change here. We are simply writing the code in a different way and in a different order.
The concept is simple but the advantages we get out of it are tremendous. Following are some of the advantages of writing code in this way.
Every Entity is Cohesive
One of the golden rules of programming is that every entity in the code should do only one thing. And this technique precisely forces us to implement just that.
We say a method is strongly cohesive if the method accomplishes one single functional aspect of overall responsibility. Single-tasking pretty much builds on the capacity of the human brain. When we multitask we are less productive and on the other hand, when we focus all our energies on a single task we complete it faster and in a more efficient way.
Programming by intention does precisely that. Every class, function, and subroutine does only one function making the code robust, clean and modular.
The code is Readable
Consider the code example I had written earlier. The concept is simple.
· A public method of a class is invoked while starting the program.
· The public method, in turn, calls two private methods.
· Each private method does only one task
· The flow is self-explanatory.
Notice that I have not included any comments in the code explaining the purpose. The flow itself is readable and easy to get to. Another thing to be noticed here is the names of the methods. The methods do exactly the way they are named. Also, since we name the methods before they actually exist, we tend to pick names that express our thinking.
We call names of this type “intention-revealing names” because they disclose the intention of the name.
And the biggest advantage of readability comes while debugging. The code is written in such a way that bugs are easier to find, track and vanquish. Since every method does only one thing, you get to the root cause of the problem much faster.
The central, organizing method in Programming by Intention(start() method in this case) contains all the steps but very little or no actual implementation. That is the key to the readable code.
Refactoring and Enhancing code becomes Easier
One of the biggest ironies of any code is that it can never be frozen. New requirements come in. Changes happen in code. Scope gets modified. The process is continuous and unavoidable.
So often we end up either refactoring the code (cleaning up a poorly written code) or enhancing the code (adding new functionality).
One of the common techniques for refactoring is the extract method. The developer takes the piece of troublesome code from the main code and creates it as a separate method having its own variables. This method after refactored is then called from the same original place. Programming by intention makes it very easy to extract as the bad code is clearly visible.
Similarly, Programming by Intention can make it easier to enhance your system. I just need to add the new functionality as a new method in the central organizing method of the code.
Nothing breaks and the functionality is modular by default and can be achieved with a high degree of confidence.
Testing Becomes Effective
Testing not only becomes effective because of the separation of different aspects of the program into individual methods. Rather programming by intention helps us in testing by allowing us to do both unit and integration testing at the same time. There are two possibilities here.
· We unit test every individual private method for the functionality intended. We know there are vulnerabilities that make them somewhat likely to fail at some point. For efficiency and security, we want to be able to test them separately from the way they are used.
· We do integrated testing through the central organizing method of the code. We execute every functional step in sequence and find out if the overall functionality is working as expected.
Note that the desire to test both aspects is forcing us to look at this “Are they really just steps?” issue, which is an important aspect of design. If this is the case, then we need to revisit our design. For example, let’s say we determine that the step that displays the data in our earlier code(display()) is not really required as part of the design, we could change the design just enough and so on…
And the biggest advantage of programming by intention is the clear visibility of failure patterns in your code. You can exactly pinpoint the failure patterns which are cropping up again and again during testing and modify the code accordingly.
And Lastly, It Brands Your Code
A lazy developer just keeps on writing lines of code as part of his daily routine. Once the day ends, he gets “lost” among the “pool” of developers who are supposed to be working on “something” every day and getting billed for the same.
On the other hand, an “active” developer makes his code unique; he brands his code with his unique signature. This “uniqueness” can be as simple as adding a “bit of code” that makes the user’s life a little easier to as complex as outstanding code optimization which boosts up the performance to significant levels.
Good Programming is a few brief moments of sublime elegance embedded in months of niggling, exacting, precise trivialities. Make that sublime elegancestand out.
Programming by intention gives you the platform to make your code stand out in all its brilliance. Your modular way of coding not only earns you accolades but also gives others a readymade platform to add further, learn more and work as a team. Together we progress towards success.
As Edsger W. Dijkstra has rightly said.
“Progress is possible only if we train ourselves to think about programs without thinking of them as pieces of executable code.”
Source: https://medium.com/swlh/1-powerful-way-to-write-robust-code-7c650071fe6b
Written by
![]()
Ravi Shankar Rajan
Medium member since Mar 2017SAP manager,Poet,Archaeology Enthusiast,History Maniac.Also a prolific writer on Leadership,Careers and Productivity at Thrive Global,Startup and The Mission
The Startup
Medium’s largest publication for makers. Subscribe to receive our top stories here → https://goo.gl/zHcLJi