You know about Arrays right? An array is a ordered collection of data. Similarly, an object is an un-ordered collection of data.
Let’s look at an array:
const justiceLeague = ['Batman', 'Superman', 'Martian Manhunter', 'Wonder Woman', 'Hawk Girl', 'Flash', 'Green Lantern'];
So I have this array (or ordered list called justiceLeague
with 7 members in in.
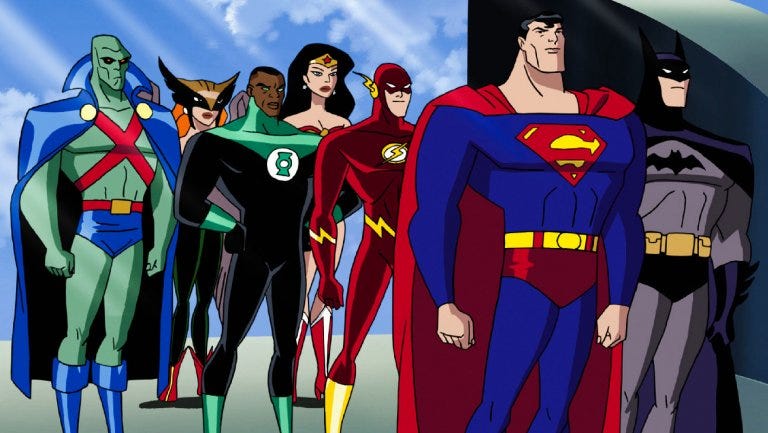
An array is an ordered list, where:
[ 0: 'Batman', 1: 'Superman', 2: 'Martian Manhunter', 3: 'Wonder Woman', 4: 'Hawk Girl', 5: 'Flash', 6: 'Green Lantern' ]
The first item is always placed at zero 0
because, binary!
So if I have to call the first member of the justice league, I will have to call justiceLeague[0]
Okay, now that you get an overview of Arrays, let’s talk about JavaScript objects.
In JavaScript, objects are typed inside curly braces {}
They are just like arrays, but they are not an ordered list.
Let’s have a look:
const crimeSyndicate = { boss: 'Superwoman', second_in_command: 'Owlman', third_in_command: 'Ultraman', sub_boss: 'Power Ring', henchman: 'Johnny Quick', second_henchman: 'White Martian', third_henchman: 'Jessica Cruz' }
So with this format, there is not first or last member, they are all designated with their respective key
‘s.
So if I were to call the boss of this object, I would do so like this: crimeSyndicate['boss']
which would return the value ‘Superwoman’.
Just like arrays, you can have sub properties of an object:
const superHero = { name: 'Barry Allen', super: { super_hero_name: 'Flash', power: 'Super Speed' } }
So if I do this: superHero['super']['power']
I will get an output of ‘Super Speed’.
There is another neat way to call this, superHero.super.power
will output the same result, this is called a dot notation.
Objects can also have functions as its members, but I will talk about it in a future post. For now, let’s wrap this up!
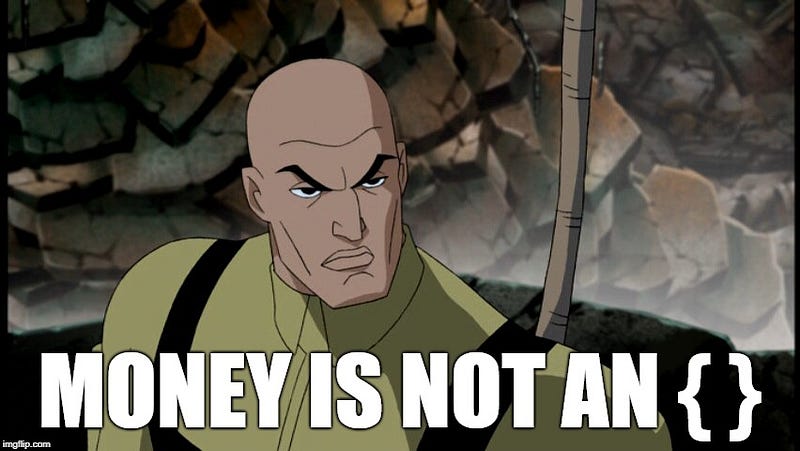